Azure.Storage.Files.Shares NuGet package makes it easy to download file(s) from Azure Storage File Share.
Using the example below, a File Share named 'content'.

Within it, there are few files and a folder named 'folder1' which contains more files.

To download the file, we need to generate the connection string to access the storage. Navigate to the Shared access signature, and specify the required access level.

In code, use ShareClient and the connection string above to initiate the connection to the Azure Storage for the specified File Share name.
Use GetRootDirectoryClient to access files in the root folder of the File Share.
Use GetDirectoryClient to access a specific folder within the File Share.
After calling Download to get the file length information, use OpenRead for access to the file content stream.
//initialise the client connection to azure storage, and the specified File Share.
var connString = "<your connection string here>";
ShareClient shareClient = new ShareClient(connString, "content");
//to access files within the root directory
ShareDirectoryClient rootDir = shareClient.GetRootDirectoryClient();
ShareFileClient sampleFile = rootDir.GetFileClient("sample150kB.pdf");
//to access files within sub directory
ShareDirectoryClient dir1 = shareClient.GetDirectoryClient("folder1");
ShareFileClient sampleFile2 = dir1.GetFileClient("sample87kB.pdf");
//retrieve the file info.
var fileDownloadInfo = sampleFile.Download().Value;
//read the file data to a stream.
var fileData = new byte[fileDownloadInfo.ContentLength];
using (var readStream = sampleFile.OpenRead(new Azure.Storage.Files.Shares.Models.ShareFileOpenReadOptions(false)))
{
readStream.Read(fileData);
}
//write to a local file.
File.WriteAllBytes("sample-downloaded.pdf", fileData);
Previously, I was using the Content object from the Download method as per below.
//there seems to be a bug here when accessing the Content. Only some file bytes are downloaded, not the entire file.
fileDownloadInfo.Content.Read(fileData, 0, fileData.Length);
File.WriteAllBytes("sample-downloaded.pdf", fileData);
There seems to be bug here that only some 3200 bytes of data downloaded, as inspected in QuickWatch.
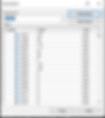
Using the OpenRead method of FileShareClient will download the entire file.
That's all for this post.